Application Architecture
An application’s architecture is more than just a single aspect of designing and developing simple or complex application systems. Many factors must be considered in order to make informed design choices for any software development project.
For web and desktop applications, we need to consider what platform the application will be hosted on. What type of web server will be used and which software libraries will be available on the hosting platform are all very important aspects of the system architecture.
For mobile applications, we need to look carefully at what types of devices and systems the application will target for distribution. Do the majority of intended users only use the latest and greatest technology? Are they predominantly iOS or Android users? All are important aspects of the design process.
Targeted Devices, Frameworks, SDKs and APIs
When we know the devices or platform the application will target, we can then select the frameworks, SDKs and libraries we will configure the application to target a specific, or minimum .NET framework version.
For mobile applications, this decision is further influenced to a great degree by application features that need to be incorporate into the application. Does the version of the API or SDK used by the application include the required portions to perform specific tasks or interact with hardware features and applications on a device? Features and device distribution both need to be considered for mobile applications.
![]() | ![]() | ![]() |
Deciding on a .NET Framework to target can be a daunting process. Portable Class Libraries might require a specific PCL Profile number, yet the feature you wish to implement might not be available, or the platform does not support that feature on older devices. Without a standard between these .NET implementations, one layer might be looking for something that is not available.
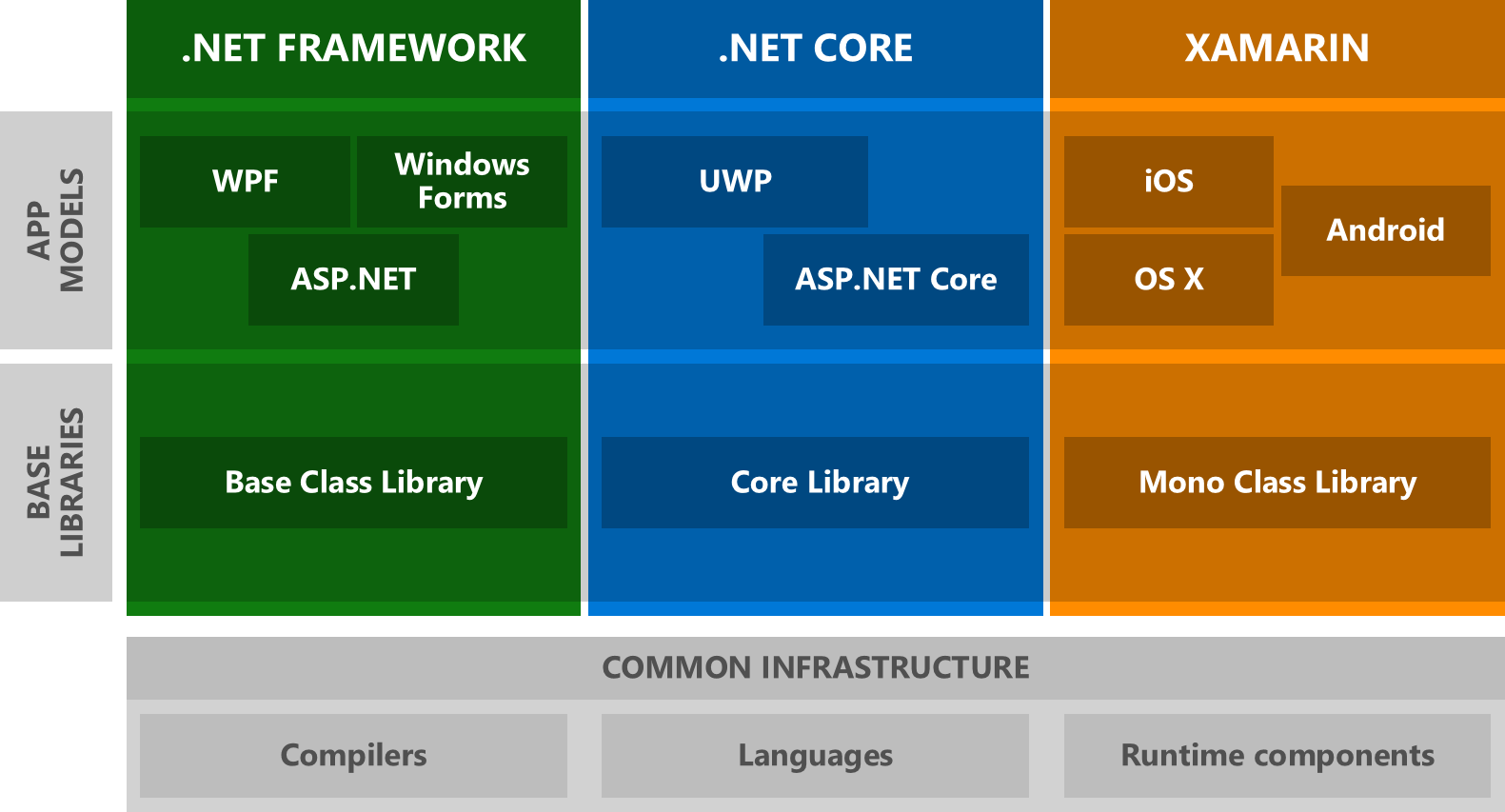
Targeting .NET Standard helps clarify and standardize how the .NET Framework operates across the various .NET implementations and environments by specifying portions supported in each .NET Standard Version. Currently, the most supported coverage is for .NET Standard 2.0.
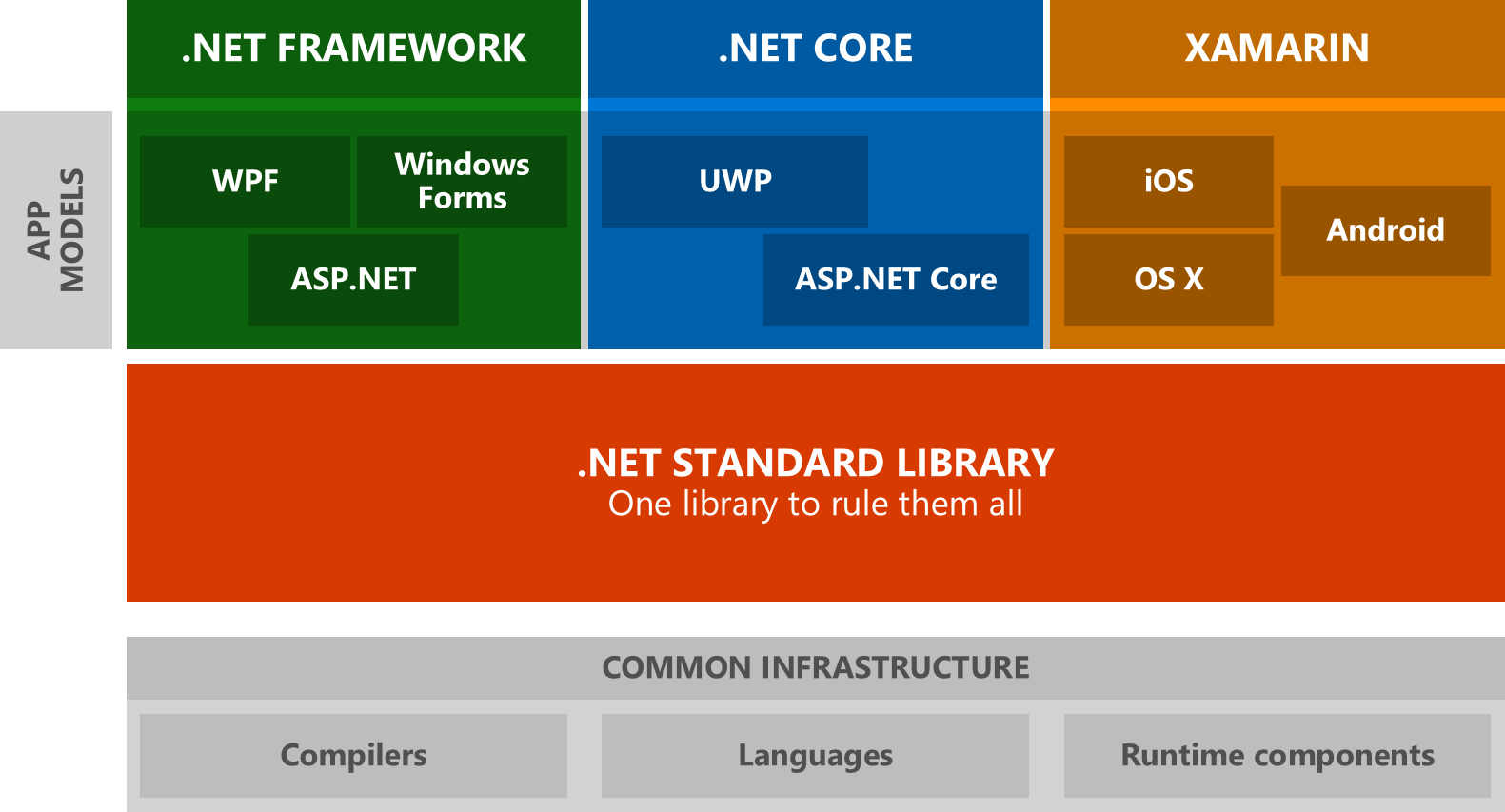
Architectural Design Patterns
Every application requires some basic architectural structure or pattern. Larger applications and enterprise level systems often require complex mixtures of architectural design patterns, but predominantly, there are three that apply to most types of software development projects. First, there is Model-View-Controller. then Model-View-Presenter and finally, Model-View-ViewModel.
Each of these patterns presents unique challenges and opportunities, and some require closer evaluation when considering mobile software applications.
Model View Controller (MVC)
MVC comes in multiple flavors these days. We have ASP.NET MVC, ASP.NET MVC Core, and then there’s just plain old MVC. At its core, MVC consists of a model, a view and a controller, as if that weren’t communicated clearly enough already. Beyond that, there’s the question of whether an MVC architecture should be an active or passive one. The actual implementation of MVC can differ significantly between platforms, but it can also differ significantly between projects or applications for the same platform.
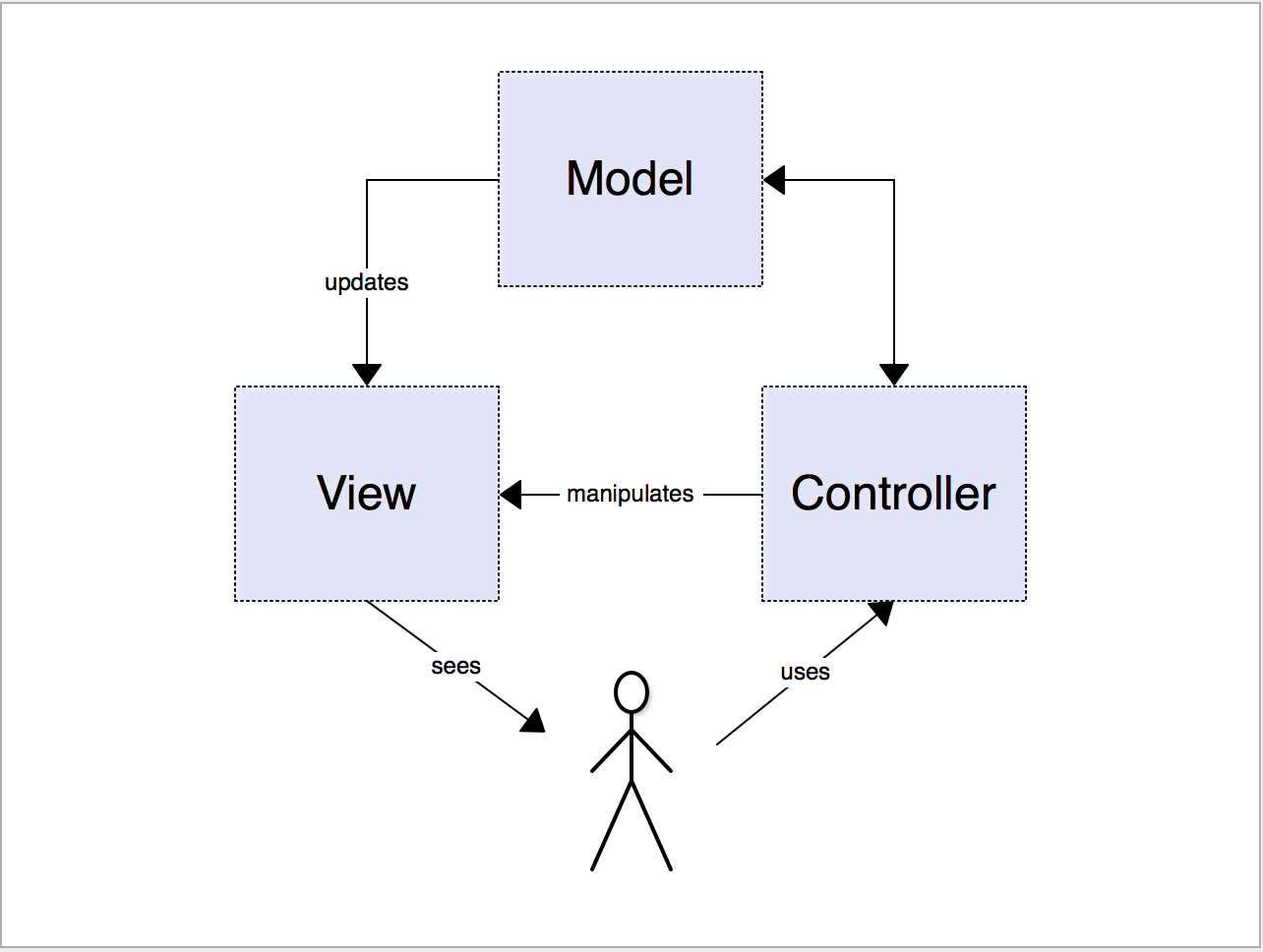
An active MVC architecture will usually implement some type of observer into the mix and use it to provide notifications to the view and controller when things change in the model.
Different implementations vary this design, sometimes significantly. In the case with producing platform, or device-specific application versions, careful thought must be applied at the beginning of a project if predominant portions of the UI are to be created natively.
This is to say that in iOS projects, they will be coded within the Xamarin.iOS libraries and SDKs, and Android projects, they will be coded within the Xamarin.Android libraries and SDKs.
While iOS might work well with MVC, Android is more inclined towards an MVP approach. Xamarin.Forms lends itself quite easily towards MVVM. Which design architecture is right for your project? Well, like most answers to questions like that is, it depends.
ASP.NET MVC and ASP.NET MVC Core are not the same platform and specific portions of the MVC design pattern might not be identical in implementation. In fact, differences in implementation should be considered to be the rule rather than exception.
Applications targeting iOS use an Apple variant of MVC. Apple takes a slightly different approach to MVC than most, and it must be considered when developing mobile applications for the iOS platform.
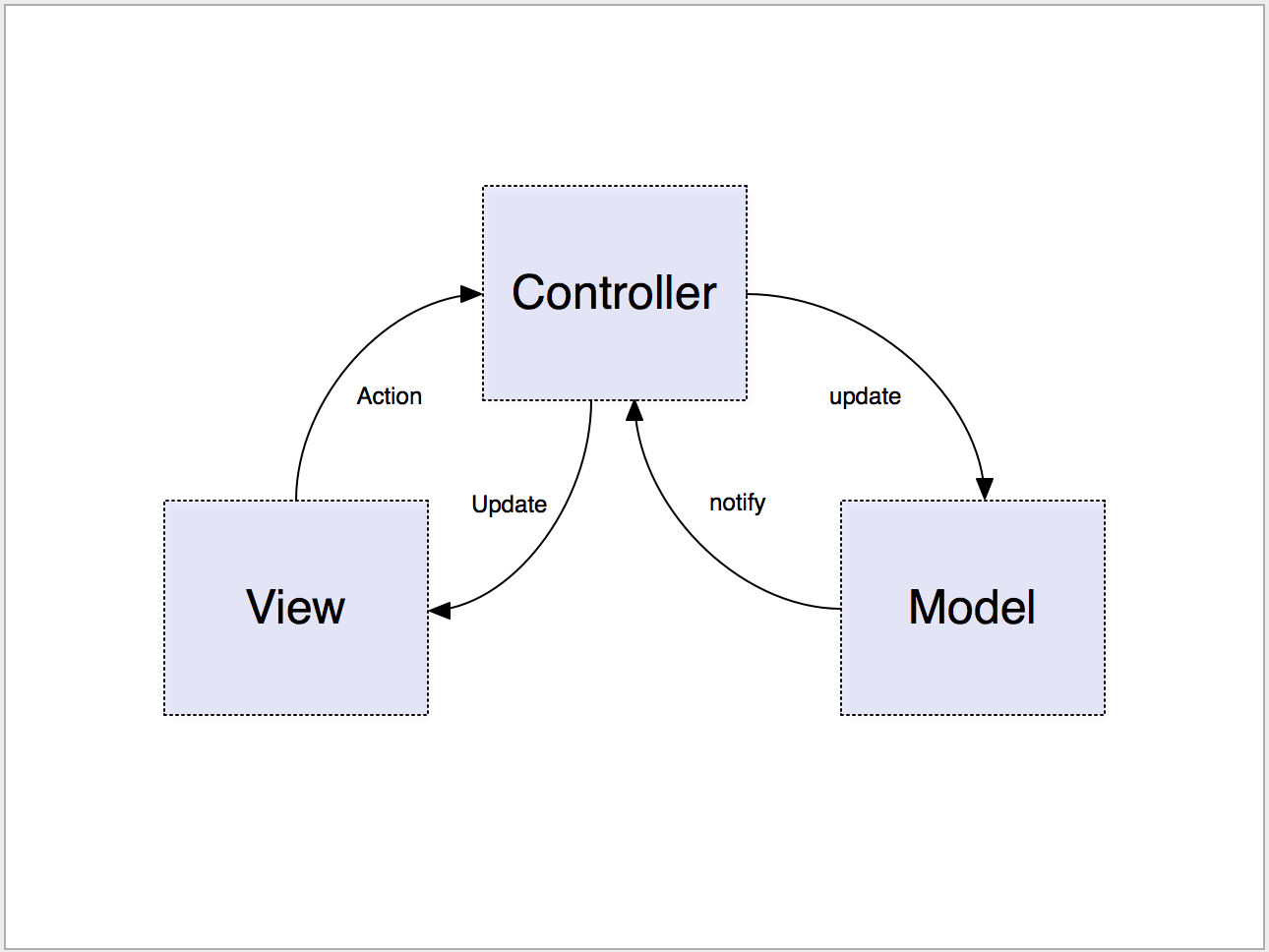
Model View Present (MVP)
Model View Presenter is a middle-man pattern that provides a layer of separation between the model and the view. The view only has knowledge of the presenter, and the model also only has knowledge of the presenter. The presenter acts as a middle man and handles interactions between the view and the model.
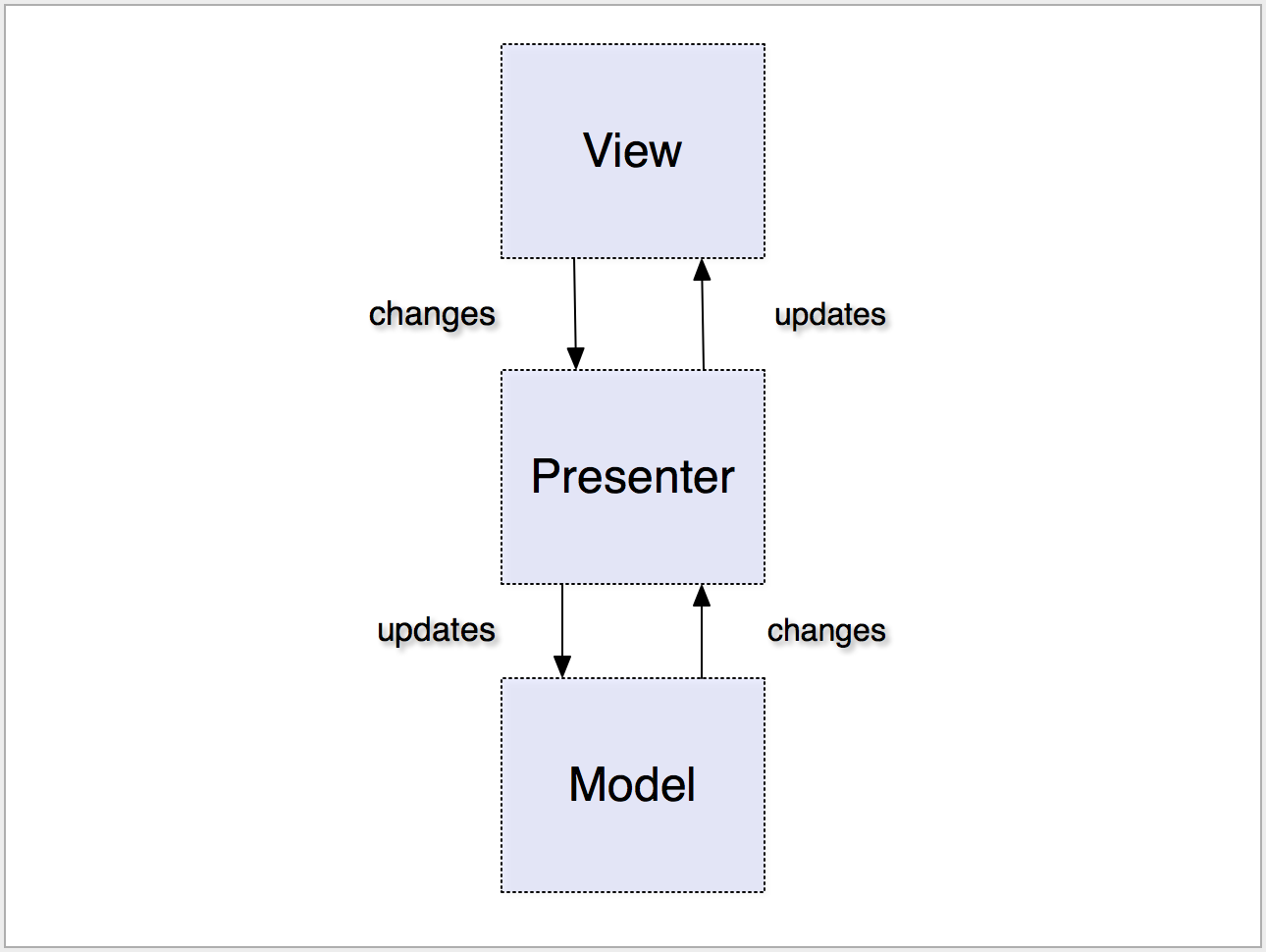
Android applications utilize MVP to separate business logic from the UI.
Model View View Model (MVVM)
The Model View ViewModel approach creates a separation between the model and view by wrapping specific properties of the model that are used in the view in a class that can also be used to provide a location for transitory variables and properties. These values are important to the view, but not to the model. We would not, for instance, persist an IsDirty or IsNew property value when it might only be used in the View Model class to determine how to display or whether to save changes to property values.
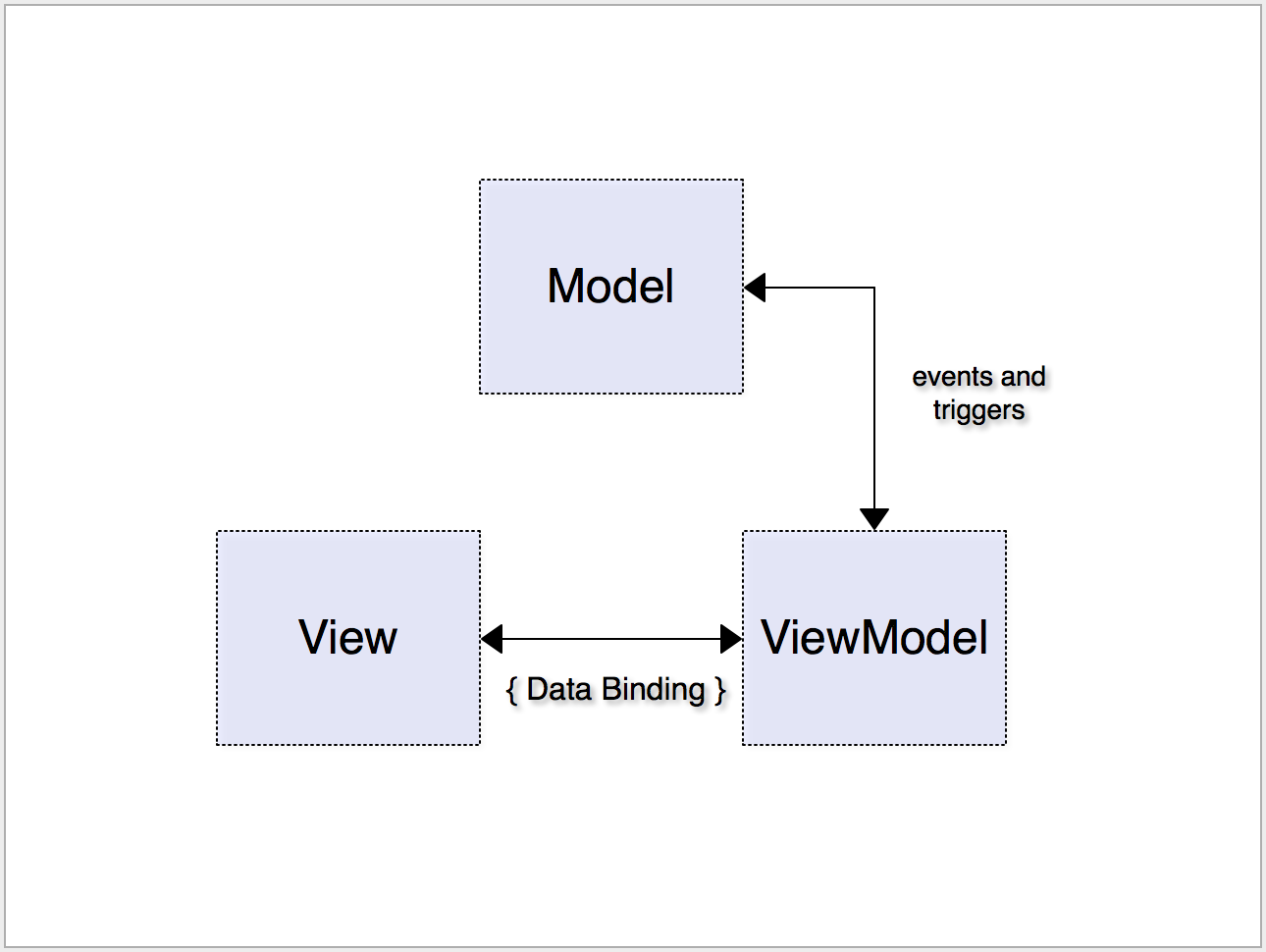
In smaller applications, MVVM can be quite easily implemented using hand-coded classes to manage the interactions between the view model, view and model layers, but in larger and more complex applications, it might be advantageous to take advantage of several MVVM libraries or frameworks.
MVVMCross (MVVM)
Just because a mobile platform might seem restrictive to one design patten, or another, MVVM can still be used with them so long as you get the internals coded correctly. Some third party libraries already exist that handle this for you. One such library is MVVMCross. There are others, but this one is really popular at the moment. The UI resides in each platform-specific project, and an MVVMCross Core library handles the interoperation between layers.
The project is located here. Project source code can be found here.
Xamarin Forms (MVVM)
There are multiple way we can implement MVVM and Xamarin Forms lends itself extremely well towards MVVM implementation; especially when using data binding of XAML controls directly to View Model properties. The ViewModel has access to a service layer that interfaces with the model layer. This separation, between the view and the model layer, enables a larger portion of applications to be testable.
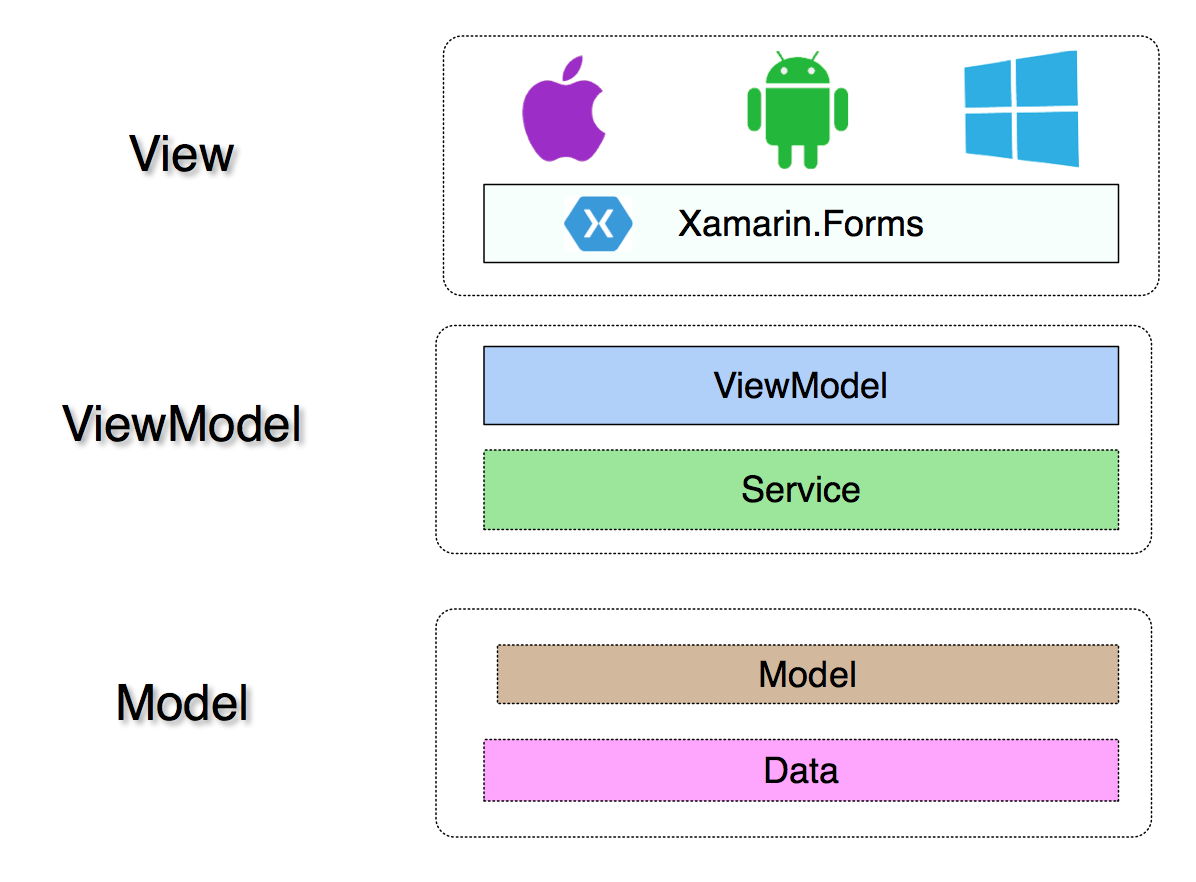
Which Design is Right for My Project
So, how do we decide which approach is the right approach for most projects? As always, it depends on many factors discovered in a requirements gathering process where we determine the target demographics, devices and features of an application, then evaluate our options and decide on an approach to designing the software.